LMC Maps
LMC Maps is a service by LMC company providing web maps based on OpenStreetMaps data.
Maps could be used on client webpages in a several ways:
- Embedded in an IFrame
- Via JavaScript library (recommended)
- As a static bitmap image
- In pure Mapbox GL JavaScript library
Embed in an Iframe
Include LMC Map to your sites or application via HTML iframe.
<iframe src="https://maps.lmc.cz/map.html?lng=14.4655&lat=50.1045&zoom=14&hasMarker&hasInteractivePois&auth_token=YOUR_TOKEN"></iframe>
Parameters
Supported languages: cs (Czech), de (German), en (English), fi (Finnish), pl (Polish), sk (Slovak)
JavaScript library
We provide client library, which is a recommended way how to use LMC Maps on a webpage. This library is built on top of Mapbox GL JS to which it adds some extra features a default configuration.
Full library documentation is available in its README.
Library can be loaded to a webpage either as a npm package or it can be included directly to HTML page via our "CDN".
Install as npm package
You can install @lmc-eu/lmc-maps
package using NPM or Yarn package managers. For more installation instructions see README of the NPM package itself.
Insert into HTML (load library from CDN)
Include JS and CSS files in the HTML file.
Place JS scripts at bottom of body tag and styles to head tag:
<!-- CSS -->
<link href="https://cdnjs.cloudflare.com/ajax/libs/mapbox-gl/1.4.0/mapbox-gl.css" rel="stylesheet" />
<link href="https://maps.lmc.cz/lib/latest/lmc-maps.css" rel="stylesheet" />
<!-- JS -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/mapbox-gl/1.4.0/mapbox-gl.js"></script>
<script src="https://maps.lmc.cz/lib/latest/lmc-maps.iife.js"></script>
Create container for map:
<div id="mapId" class="map" style="width: 600px; height: 360px;"></div>
Initialize LMC Map instance:
new LmcMaps({
container: 'mapContainer',
zoom: 7,
center: [15.524, 49.766],
coords: [[15.524, 49.766], [15.481, 49.758]],
hasMarker: true,
hasInteractivePois: true,
lang: 'cs',
// Tileserver running on default publicUrl require use of authToken. For demonstration and development purposes only, you can however override default publicUrl to use our "demo tileserver":
publicUrl: 'https://tileserver.lmc.cz/demo' // DO NOT USE demo tileserver on production! (It has low rate limit.)
// authToken is not needed for "demo tileserver" but must be defined for production use:
// authToken: 'YOUR_TOKEN' // DO NOT FORGET to define auth token for production use
});
Configuration
See reference documentation on GitHub.
Example
<!DOCTYPE html>
<html>
<head>
<title>Map</title>
<link href="https://cdnjs.cloudflare.com/ajax/libs/mapbox-gl/1.12.0/mapbox-gl.css" rel="stylesheet" />
<link href="https://maps.lmc.cz/lib/latest/lmc-maps.css" rel="stylesheet" />
</head>
<body>
<div id="mapContainer" class="map" style="width: 600px; height: 360px;"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/mapbox-gl/1.12.0/mapbox-gl.js"></script>
<script src="https://maps.lmc.cz/lib/latest/lmc-maps.iife.js"></script>
<script>
(function() {
new LmcMaps({
container: 'mapContainer',
zoom: 7,
center: [15.524, 49.766],
coords: [[15.524, 49.766], [15.481, 49.758]],
hasMarker: true,
hasInteractivePois: true,
lang: 'cs',
publicUrl: 'https://tileserver.lmc.cz/demo' // DO NOT USE demo tileserver on production! (It has low rate limit.)
});
})();
</script>
</body>
</html>
Static Maps
Using tileserver for rendering static map as image. Define GL style, coordinates, zoom and size of generated image. LMC Authorization Token in request header is required.
https://tileserver.lmc.cz/styles/{STYLE}/static/{LONGITUDE},{LATITUDE},{ZOOM}/{WIDTH}x{HEIGHT}.{FORMAT}
Example of request:
curl -H "Authorization: Bearer ---PLACE_LMC_AUTH_TOKEN_HERE---" -o staticMap.png https://tileserver.lmc.cz/styles/lmc-default/static/14.4655,50.1045,10/1200x600.png
Example static map
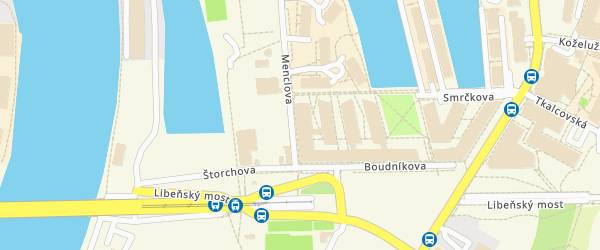
This map uses demo tileserver (with low rate limit), you must use your own token for production use.
Options
Using directly in Mapbox GL JS application
LMC Maps could be used directly through Mapbox GL JS library (i.e. without LMC Maps JavaScript library). Even though this is not recommended, it is possible by including selected GL style in your Mapbox GL JS application:
Mapbox Authorization Token is NOT required, but you have to add LMC Authorization Token for LMC Tiles.
const map = new mapboxgl.Map({
container: 'mapId',
style: 'https://tileserver.lmc.cz/styles/lmc-default/style.json',
center: [15.524, 49.766],
zoom: 10,
transformRequest: (url, resourceType) => {
if (resourceType === 'Tile') {
return {
url: url,
headers: {
'Authorization': 'Bearer ---PLACE_LMC_AUTH_TOKEN_HERE---'
}
}
}
}
});
Find more information at OpenMapTiles.org.
Map styles
We provide lmc-default
map style with custom colors, layers etc. However other MapBox GL styles can be used, for example klokantech-basic
.